Espressif 32
- Registry:
https://registry.platformio.org/platforms/platformio/espressif32
- Configuration:
platform =
platformio/espressif32
ESP32 is a series of low-cost, low-power system on a chip microcontrollers with integrated Wi-Fi and Bluetooth. ESP32 integrates an antenna switch, RF balun, power amplifier, low-noise receive amplifier, filters, and power management modules.
For more detailed information please visit vendor site.
Tutorials
Configuration
CPU Frequency
See board_build.f_cpu option from “platformio.ini” (Project Configuration File)
[env:myenv]
; set frequency to 160MHz
board_build.f_cpu = 160000000L
FLASH Frequency
Please use board_build.f_flash
option from “platformio.ini” (Project Configuration File) to change
a value. Possible values:
40000000L
(default)80000000L
[env:myenv]
; set frequency to 80MHz
board_build.f_flash = 80000000L
FLASH Mode
Flash chip interface mode. This parameter is stored in the binary image header, along with the flash size and flash frequency. The ROM bootloader in the ESP chip uses the value of these parameters in order to know how to talk to the flash chip.
Please use board_build.flash_mode
option from “platformio.ini” (Project Configuration File) to change
a value. Possible values:
qio
qout
dio
dout
[env:myenv]
board_build.flash_mode = qio
External RAM (PSRAM)
You can enable external RAM using the next extra build_flags in “platformio.ini” (Project Configuration File) depending on a framework type.
Framework Arduino:
[env:myenv]
platform = platformio/espressif32
framework = arduino
board = ...
build_flags =
-DBOARD_HAS_PSRAM
-mfix-esp32-psram-cache-issue
Framework Espressif IoT Development Framework:
[env:myenv]
platform = platformio/espressif32
framework = espidf
board = ...
build_flags =
-DCONFIG_SPIRAM_CACHE_WORKAROUND
More details are located in the official ESP-IDF documentation - Support for external RAM.
Debug Level
Please use one of the next build_flags to change debug level. A build_flags option could be used only the one time per build environment. If you need to specify more flags, please separate them with a new line or space.
Actual information is available in Arduino for ESP32 Board Manifest.
Please scroll to esp32.menu.DebugLevel
section.
[env:myenv]
platform = ...
board = ...
framework = arduino
;;;;; Possible options ;;;;;;
; None
build_flags = -DCORE_DEBUG_LEVEL=0
; Error
build_flags = -DCORE_DEBUG_LEVEL=1
; Warn
build_flags = -DCORE_DEBUG_LEVEL=2
; Info
build_flags = -DCORE_DEBUG_LEVEL=3
; Debug
build_flags = -DCORE_DEBUG_LEVEL=4
; Verbose
build_flags = -DCORE_DEBUG_LEVEL=5
Upload Speed
You can set custom upload speed using upload_speed option from “platformio.ini” (Project Configuration File)
[env:myenv]
upload_speed = 9600
Erase Flash
Please pio run --target
the next command to erase the entire
flash chip (all data replaced with 0xFF bytes):
> pio run --target erase
# or short version
> pio run -t erase
Partition Tables
You can create a custom partitions table (CSV) following ESP32 Partition Tables documentation. PlatformIO uses default partition tables depending on a framework type:
default.csv
for Arduino (show pre-configured partition tables)partitions_singleapp.csv
for Espressif IoT Development Framework (show pre-configured partition tables)
To override default table please use board_build.partitions
option in
“platformio.ini” (Project Configuration File).
Warning
SPIFFS partition MUST have configured “Type” as “data” and “SubType”
as “spiffs”. For example, spiffs, data, spiffs, 0x291000, 1M,
Examples:
; 1) A "partitions_custom.csv" in the root of project directory
[env:custom_table]
board_build.partitions = partitions_custom.csv
; 2) Switch between built-in tables
; https://github.com/espressif/arduino-esp32/tree/master/tools/partitions
; https://github.com/espressif/esp-idf/tree/master/components/partition_table
[env:custom_builtin_table]
board_build.partitions = no_ota.csv
Embedding Binary Data
Sometimes you have a file with some binary or text data that you’d like to make available to your program - but you don’t want to reformat the file as C source.
There are two options board_build.embed_txtfiles
and board_build.embed_files
which can be used for embedding data. The only difference is that files specified
in board_build.embed_txtfiles
option are null-terminated in the final binary.
[env:myenv]
platform = platformio/espressif32
board = ...
board_build.embed_txtfiles =
src/private.pem.key
src/certificate.pem.crt
src/aws-root-ca.pem
Note
In case with ESP-IDF, these files also need to be specified in CMakeLists.txt
using the target_add_binary_data
function.
The file contents will be added to the .rodata
section in flash, and
are available via symbol names as follows:
extern const uint8_t aws_root_ca_pem_start[] asm("_binary_src_aws_root_ca_pem_start");
extern const uint8_t aws_root_ca_pem_end[] asm("_binary_src_aws_root_ca_pem_end");
extern const uint8_t certificate_pem_crt_start[] asm("_binary_src_certificate_pem_crt_start");
extern const uint8_t certificate_pem_crt_end[] asm("_binary_src_certificate_pem_crt_end");
extern const uint8_t private_pem_key_start[] asm("_binary_src_private_pem_key_start");
extern const uint8_t private_pem_key_end[] asm("_binary_src_private_pem_key_end");
The names are generated from the full name of the file. Characters /, .
,
etc. are replaced with underscores. The _binary
+ _nested_folder
prefix
in the symbol name is added by “objcopy” and is the same for both text and binary files.
Note
With the ESP-IDF framework symbol names should not contain path to the files, for example
_binary_private_pem_key_start
instead of _binary_src_private_pem_key_start
.
See full example with embedding Amazon AWS certificates:
Uploading files to file system
Create new project using PlatformIO IDE or initialize project using PlatformIO Core (CLI) and pio project init (if you have not initialized it yet)
Create
data
folder (it should be on the same level assrc
folder) and put files here. Also, you can specify own location for data_dirRun “Upload File System image” task in PlatformIO IDE or use PlatformIO Core (CLI) and
pio run --target
command withuploadfs
target.
The SPIFFS
file system is used by default in order to keep legacy project
compatible. To choose LittleFS
or FFat
as the file system, it should be
explicitly specified using board_build.filesystem
option in “platformio.ini” (Project Configuration File),
for example:
[env:myenv]
platform = platformio/espressif32
framework = arduino
board = ...
board_build.filesystem = littlefs
Possible values for board_build.filesystem
are spiffs
(default), littlefs
and fatfs
.
To upload SPIFFS image using OTA update please specify upload_port
/
--upload-port
as IP address or mDNS host name (ending with the *.local
).
Examples:
Over-the-Air (OTA) update
Warning
Please make sure to read the theory behind the OTA updates in the Over The Air Updates (OTA) article.
Create a new project using PlatformIO Home or initialize a project via PlatformIO Core (CLI) and pio project init (if you have not initialized it yet)
Copy the basicOTA example for Arduino or Simple OTA example for ESP-IDF to src_dir and configure your WiFi credentials (SSID and password).
Compile the project to ensure there are no syntax errors in the code.
To upload the binary you can either specify the upload address directly in the CLI
command using the pio run --upload-port
option:
pio run --target upload --upload-port IP_ADDRESS_HERE or mDNS_NAME.local
For example:
pio run -t upload --upload-port 192.168.0.255
pio run -t upload --upload-port myesp32.local
Or use the upload_port
option in “platformio.ini” (Project Configuration File). Please note that you also
need to set upload_protocol to espota
:
[env:myenv]
upload_protocol = espota
upload_port = IP_ADDRESS_HERE or mDNS_NAME.local
For example:
[env:myenv]
platform = platformio/espressif32
board = esp32dev
framework = arduino
upload_protocol = espota
upload_port = 192.168.0.255
Authentication and upload options
You can pass additional options/flags to OTA uploader using
upload_flags
option in “platformio.ini” (Project Configuration File)
[env:myenv]
upload_protocol = espota
; each flag in a new line
upload_flags =
--port=3232
Available flags
--port=ESP_PORT
ESP32 OTA Port. Default 8266--auth=AUTH
Set authentication password--spiffs
Use this option to transmit a SPIFFS image and do not flash the module
For the full list with available options please run
~/.platformio/packages/framework-arduinoespressif32/tools/espota.py --help
Usage: espota.py [options]
Transmit image over the air to the esp32 module with OTA support.
Options:
-h, --help show this help message and exit
Destination:
-i ESP_IP, --ip=ESP_IP
ESP32 IP Address.
-I HOST_IP, --host_ip=HOST_IP
Host IP Address.
-p ESP_PORT, --port=ESP_PORT
ESP32 ota Port. Default 3232
-P HOST_PORT, --host_port=HOST_PORT
Host server ota Port. Default random 10000-60000
Authentication:
-a AUTH, --auth=AUTH
Set authentication password.
Image:
-f FILE, --file=FILE
Image file.
-s, --spiffs Use this option to transmit a SPIFFS image and do not
flash the module.
Output:
-d, --debug Show debug output. And override loglevel with debug.
-r, --progress Show progress output. Does not work for ArduinoIDE
-t TIMEOUT, --timeout=TIMEOUT
Timeout to wait for the ESP32 to accept invitation
Warning
For windows users. To manage OTA check the ESP wifi network profile isn’t checked on public be sure it’s on private mode``
Using Arduino Framework with Staging version
PlatformIO will install the latest Arduino Core for ESP32 from
https://github.com/espressif/arduino-esp32. The Git
should be installed in a system. To update Arduino Core to the latest revision,
please open PlatformIO IDE and navigate to PlatformIO Home > Platforms > Updates
.
Please install PlatformIO IDE
Initialize a new project, open “platformio.ini” (Project Configuration File) and specify the link to the framework repository in platform_packages section. For example,
[env:esp32dev] platform = platformio/espressif32 board = esp32dev framework = arduino platform_packages = platformio/framework-arduinoespressif32 @ https://github.com/espressif/arduino-esp32.git
Try to build the project
If you see build errors, then try to build this project using the same
stage
with Arduino IDEIf it works with Arduino IDE but doesn’t work with PlatformIO, then please file a new issue with attached information:
test project/files
detailed log of build process from Arduino IDE (please copy it from console to https://hastebin.com)
detailed log of build process from PlatformIO Build System (please copy it from console to https://hastebin.com)
Arduino Core Wiki
Tips, tricks and common problems: http://desire.giesecke.tk/index.php/2018/01/30/esp32-wiki-entries/
Examples
Examples are listed from Espressif 32 development platform repository:
Debugging
Debugging - “1-click” solution for debugging with a zero configuration.
Pinout Diagram
JTAG Wiring Connections
Board Pin |
JTAG Tool Pin |
---|---|
IO13 |
TCK |
IO12 |
TDI |
IO15 |
TDO |
IO14 |
TMS |
EN |
RST |
GND |
GND |
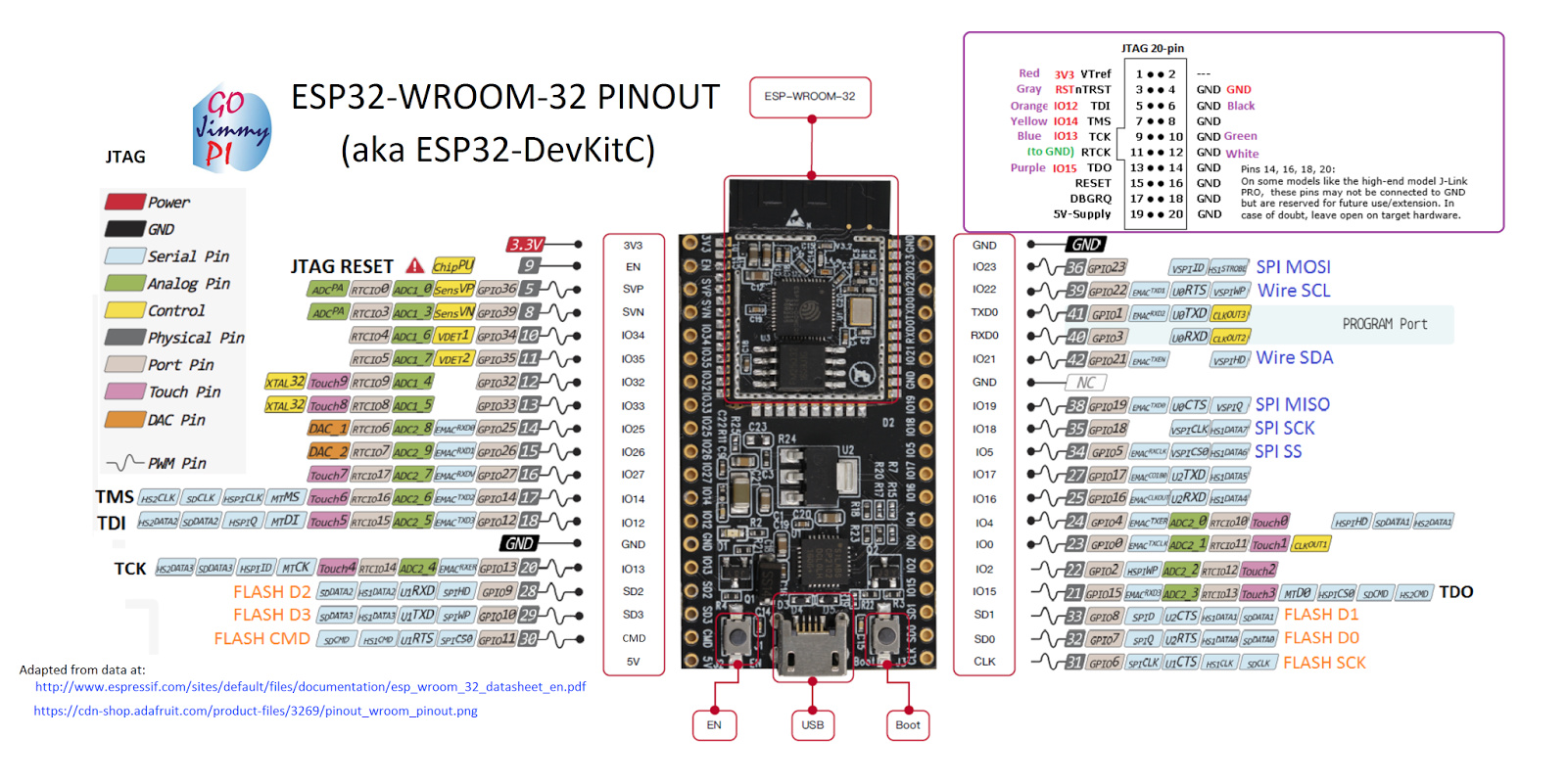
Tools & Debug Probes
Supported debugging tools are listed in “Debug” column. For more detailed information, please scroll table by horizontal. You can switch between debugging Tools & Debug Probes using debug_tool option in “platformio.ini” (Project Configuration File).
Warning
You will need to install debug tool drivers depending on your system. Please click on compatible debug tool below for the further instructions.
Boards listed below have on-board debug probe and ARE READY for debugging! You do not need to use/buy external debug probe.
Name |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32S2 |
240MHz |
4MB |
320KB |
|
ESP32S3 |
240MHz |
8MB |
320KB |
|
ESP32S3 |
240MHz |
8MB |
320KB |
|
ESP32S3 |
240MHz |
8MB |
320KB |
|
ESP32S3 |
240MHz |
8MB |
320KB |
|
ESP32S3 |
240MHz |
4MB |
320KB |
|
ESP32S3 |
240MHz |
8MB |
320KB |
Boards listed below are compatible with Debugging but DEPEND ON external debug probe. They ARE NOT READY for debugging. Please click on board name for the further details.
Name |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|
ESP32S3 |
240MHz |
16MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
520KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32S2 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
8MB |
320KB |
|
ESP32S2 |
240MHz |
4MB |
320KB |
|
ESP32S2 |
240MHz |
4MB |
320KB |
|
ESP32S2 |
240MHz |
4MB |
320KB |
|
ESP32S3 |
240MHz |
4MB |
320KB |
|
ESP32S3 |
240MHz |
8MB |
320KB |
|
ESP32S3 |
240MHz |
4MB |
320KB |
|
ESP32S3 |
240MHz |
4MB |
320KB |
|
ESP32S2 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
8MB |
320KB |
|
ESP32S2 |
240MHz |
4MB |
320KB |
|
ESP32S3 |
240MHz |
8MB |
320KB |
|
ESP32S2 |
240MHz |
4MB |
320KB |
|
ESP32S3 |
240MHz |
16MB |
320KB |
|
ESP32 |
240MHz |
8MB |
320KB |
|
ESP32C3 |
160MHz |
4MB |
320KB |
|
ESP32S2 |
240MHz |
4MB |
320KB |
|
ESP32S3 |
240MHz |
4MB |
320KB |
|
ESP32S3 |
240MHz |
8MB |
320KB |
|
ESP32S3 |
240MHz |
16MB |
320KB |
|
ESP32S3 |
240MHz |
4MB |
320KB |
|
ESP32C3 |
160MHz |
4MB |
320KB |
|
ESP32S2 |
240MHz |
4MB |
320KB |
|
ESP32C3 |
160MHz |
4MB |
320KB |
|
ESP32S3 |
240MHz |
16MB |
320KB |
|
ESP32S3 |
240MHz |
8MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32S3 |
240MHz |
16MB |
320KB |
|
ESP32S3 |
240MHz |
8MB |
320KB |
|
ESP32S3 |
240MHz |
8MB |
320KB |
|
ESP32C3 |
160MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32S3 |
240MHz |
8MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32C3 |
160MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32S3 |
240MHz |
4MB |
320KB |
|
ESP32S3 |
240MHz |
16MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32S3 |
240MHz |
4MB |
320KB |
|
ESP32C3 |
160MHz |
4MB |
320KB |
|
ESP32S2 |
240MHz |
4MB |
320KB |
|
ESP32S2 |
240MHz |
4MB |
320KB |
|
ESP32S2 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32S3 |
240MHz |
8MB |
320KB |
|
ESP32S3 |
240MHz |
8MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32S2 |
240MHz |
16MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32C3 |
160MHz |
4MB |
320KB |
|
ESP32C3 |
160MHz |
4MB |
320KB |
|
ESP32C6 |
160MHz |
4MB |
320KB |
|
ESP32S2 |
240MHz |
4MB |
320KB |
|
ESP32S3 |
240MHz |
16MB |
320KB |
|
ESP32 |
240MHz |
16MB |
520KB |
|
ESP32S2 |
240MHz |
4MB |
320KB |
|
ESP32S2 |
240MHz |
4MB |
320KB |
|
ESP32S2 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32S3 |
240MHz |
8MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
8MB |
320KB |
|
ESP32S3 |
240MHz |
8MB |
320KB |
|
ESP32 |
240MHz |
8MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32S3 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32S3 |
240MHz |
16MB |
320KB |
|
ESP32S3 |
240MHz |
16MB |
320KB |
|
ESP32S3 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32S3 |
240MHz |
8MB |
320KB |
|
ESP32S3 |
240MHz |
16MB |
320KB |
|
ESP32S3 |
240MHz |
8MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32S3 |
240MHz |
4MB |
320KB |
|
ESP32S3 |
240MHz |
8MB |
320KB |
|
ESP32S3 |
240MHz |
4MB |
320KB |
|
ESP32S3 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
1.25MB |
|
ESP32 |
240MHz |
4MB |
1.25MB |
|
ESP32C3 |
160MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32S3 |
240MHz |
4MB |
320KB |
|
ESP32C3 |
160MHz |
4MB |
320KB |
|
ESP32S3 |
240MHz |
8MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32S3 |
240MHz |
8MB |
320KB |
|
ESP32S2 |
240MHz |
4MB |
320KB |
|
ESP32C3 |
160MHz |
4MB |
320KB |
|
ESP32S3 |
240MHz |
8MB |
320KB |
|
ESP32S3 |
240MHz |
8MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
16MB |
320KB |
|
ESP32 |
240MHz |
16MB |
320KB |
|
ESP32S2 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
8MB |
320KB |
|
ESP32S3 |
240MHz |
8MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
1.25MB |
|
ESP32C3 |
160MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
1.25MB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32S2 |
240MHz |
16MB |
320KB |
|
ESP32S2 |
240MHz |
4MB |
320KB |
|
ESP32S3 |
240MHz |
16MB |
320KB |
|
ESP32S3 |
240MHz |
8MB |
320KB |
|
ESP32S3 |
240MHz |
16MB |
320KB |
|
ESP32S2 |
240MHz |
4MB |
320KB |
|
ESP32S2 |
240MHz |
4MB |
320KB |
|
ESP32S3 |
240MHz |
8MB |
320KB |
|
ESP32C3 |
160MHz |
4MB |
320KB |
|
ESP32C3 |
160MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32C3 |
160MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32S2 |
240MHz |
4MB |
320KB |
|
ESP32S2 |
240MHz |
4MB |
320KB |
|
ESP32S3 |
240MHz |
16MB |
320KB |
|
ESP32S3 |
240MHz |
4MB |
320KB |
|
ESP32S3 |
240MHz |
16MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32C3 |
160MHz |
384KB |
400KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32S2 |
240MHz |
16MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32S2 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32 |
240MHz |
4MB |
320KB |
|
ESP32S3 |
240MHz |
7.94MB |
2.31MB |
|
ESP32S3 |
240MHz |
7.94MB |
8.31MB |
Stable and upstream versions
You can switch between stable releases of Espressif 32 development platform and the latest upstream version using platform option in “platformio.ini” (Project Configuration File) as described below.
Stable
; Latest stable version, NOT recommended
; Pin the version as shown below
[env:latest_stable]
platform = espressif32
board = ...
; Specific version
[env:custom_stable]
platform = espressif32@x.y.z
board = ...
Upstream
[env:upstream_develop]
platform = https://github.com/platformio/platform-espressif32.git
board = ...
Packages
Name |
Description |
---|---|
Arduino Wiring-based Framework for the Espressif ESP32, ESP32-S and ESP32-C series of SoCs |
|
Espressif IoT Development Framework. Official development framework for ESP32 chip |
|
CMake is an open-source, cross-platform family of tools designed to build, test and package software |
|
Device Firmware Upgrade Utilities |
|
A Python-based, open-source, platform-independent utility to communicate with the ROM bootloader in Espressif chips |
|
idf is a top-level config/build command line tool for ESP-IDF |
|
Fork of kconfig-frontends project with some modifications for use with ESP-IDF |
|
Utility for creating FFat images |
|
Utility for creating littlefs images for upload on the ESP8266 |
|
Tool to build and unpack SPIFFS images |
|
Ninja is a small build system with a focus on speed |
|
Open On-Chip Debugger for Espressif ESP32 |
|
GNU GDB for Espressif ESP32 Xtensa MCUs (RISC-V Core) |
|
GNU GDB for Espressif ESP32 Xtensa MCUs (RISC-V Core) |
|
Binutils fork with support for the Espressif ESP32 ULP co-processor |
|
GCC Toolchain for Espressif 32-bit RISC-V based MCUs |
|
GCC Toolchain for Espressif ESP32 Xtensa MCUs |
|
GCC Toolchain for Espressif ESP32 Xtensa MCUs |
|
GCC Toolchain for Espressif ESP32-S2 Xtensa MCUs |
|
GCC Toolchain for Espressif ESP32-S3 Xtensa MCUs |
Warning
Linux Users:
Install “udev” rules 99-platformio-udev.rules
Raspberry Pi users, please read this article Enable serial port on Raspberry Pi.
Windows Users:
Please check that you have a correctly installed USB driver from board manufacturer
Frameworks
Name |
Description |
---|---|
Arduino Wiring-based Framework allows writing cross-platform software to control devices attached to a wide range of Arduino boards to create all kinds of creative coding, interactive objects, spaces or physical experiences. |
|
Espressif IoT Development Framework. Official development framework for ESP32 chip |
Boards
Note
You can list pre-configured boards by pio boards command
For more detailed
board
information please scroll the tables below by horizontally.
4D Systems
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32S3 |
240MHz |
16MB |
320KB |
AI Thinker
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32 |
240MHz |
4MB |
320KB |
AZ-Delivery
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32 |
240MHz |
4MB |
520KB |
Adafruit
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32 |
240MHz |
4MB |
320KB |
|
External |
ESP32S2 |
240MHz |
4MB |
320KB |
|
External |
ESP32 |
240MHz |
8MB |
320KB |
|
External |
ESP32S2 |
240MHz |
4MB |
320KB |
|
External |
ESP32S2 |
240MHz |
4MB |
320KB |
|
External |
ESP32S2 |
240MHz |
4MB |
320KB |
|
External |
ESP32S3 |
240MHz |
4MB |
320KB |
|
External |
ESP32S3 |
240MHz |
8MB |
320KB |
|
External |
ESP32S3 |
240MHz |
4MB |
320KB |
|
External |
ESP32S3 |
240MHz |
4MB |
320KB |
|
External |
ESP32S2 |
240MHz |
4MB |
320KB |
|
External |
ESP32 |
240MHz |
8MB |
320KB |
|
External |
ESP32S2 |
240MHz |
4MB |
320KB |
|
External |
ESP32S3 |
240MHz |
8MB |
320KB |
|
External |
ESP32S2 |
240MHz |
4MB |
320KB |
|
External |
ESP32S3 |
240MHz |
16MB |
320KB |
|
External |
ESP32 |
240MHz |
8MB |
320KB |
|
External |
ESP32C3 |
160MHz |
4MB |
320KB |
|
External |
ESP32S2 |
240MHz |
4MB |
320KB |
|
External |
ESP32S3 |
240MHz |
4MB |
320KB |
|
External |
ESP32S3 |
240MHz |
8MB |
320KB |
|
External |
ESP32S3 |
240MHz |
16MB |
320KB |
|
External |
ESP32S3 |
240MHz |
4MB |
320KB |
Ai-Thinker
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32C3 |
160MHz |
4MB |
320KB |
|
External |
ESP32S2 |
240MHz |
4MB |
320KB |
AirM2M
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32C3 |
160MHz |
4MB |
320KB |
Aiyarafun
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32 |
240MHz |
4MB |
320KB |
Anderson & friends
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32S3 |
240MHz |
16MB |
320KB |
April Brother
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
No |
ESP32 |
240MHz |
4MB |
320KB |
Arduino
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32S3 |
240MHz |
16MB |
320KB |
ArtronShop
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32S3 |
240MHz |
8MB |
320KB |
|
External |
ESP32 |
240MHz |
4MB |
320KB |
|
External |
ESP32 |
240MHz |
4MB |
320KB |
Aventen
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32S3 |
240MHz |
16MB |
320KB |
BPI Tech
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
No |
ESP32 |
160MHz |
4MB |
320KB |
|
External |
ESP32S3 |
240MHz |
8MB |
320KB |
Blinker
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
No |
ESP32 |
240MHz |
4MB |
320KB |
|
External |
ESP32S3 |
240MHz |
8MB |
320KB |
|
External |
ESP32C3 |
160MHz |
4MB |
320KB |
CNRS
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
No |
ESP32 |
240MHz |
4MB |
320KB |
Connaxio
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32 |
240MHz |
4MB |
320KB |
Cytron Technologies
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32S3 |
240MHz |
8MB |
320KB |
DFRobot
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32C3 |
160MHz |
4MB |
320KB |
|
External |
ESP32 |
240MHz |
4MB |
320KB |
|
External |
ESP32S3 |
240MHz |
4MB |
320KB |
|
External |
ESP32S3 |
240MHz |
16MB |
320KB |
|
External |
ESP32 |
240MHz |
16MB |
520KB |
DOIT
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32 |
240MHz |
4MB |
320KB |
|
External |
ESP32 |
240MHz |
4MB |
320KB |
DSTIKE
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32 |
240MHz |
4MB |
320KB |
Denky
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
No |
ESP32 |
240MHz |
8MB |
320KB |
|
No |
ESP32 |
240MHz |
4MB |
320KB |
Department of Alchemy
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32S2 |
240MHz |
4MB |
320KB |
Dongsen Technology
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32 |
240MHz |
4MB |
320KB |
DycodeX
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32 |
240MHz |
4MB |
320KB |
ESP32vn
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32 |
240MHz |
4MB |
320KB |
ETBoard
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
No |
ESP32 |
240MHz |
4MB |
320KB |
Electronic SweetPeas
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
No |
ESP32 |
240MHz |
4MB |
320KB |
EspinalLab
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32S2 |
240MHz |
16MB |
320KB |
Espressif
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32 |
240MHz |
4MB |
320KB |
|
External |
ESP32S3 |
240MHz |
8MB |
320KB |
|
On-board |
ESP32 |
240MHz |
4MB |
320KB |
|
External |
ESP32 |
240MHz |
4MB |
320KB |
|
External |
ESP32C3 |
160MHz |
4MB |
320KB |
|
External |
ESP32C3 |
160MHz |
4MB |
320KB |
|
No |
ESP32C6 |
160MHz |
8MB |
320KB |
|
External |
ESP32C6 |
160MHz |
4MB |
320KB |
|
On-board |
ESP32S2 |
240MHz |
4MB |
320KB |
|
External |
ESP32S2 |
240MHz |
4MB |
320KB |
|
External |
ESP32S3 |
240MHz |
16MB |
320KB |
|
On-board |
ESP32S3 |
240MHz |
8MB |
320KB |
|
On-board |
ESP32S3 |
240MHz |
8MB |
320KB |
|
On-board |
ESP32S3 |
240MHz |
8MB |
320KB |
Espressif Systems
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
No |
ESP32 |
240MHz |
8MB |
320KB |
Fishino
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
No |
ESP32 |
240MHz |
4MB |
320KB |
Franzininho
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32S2 |
240MHz |
4MB |
320KB |
|
External |
ESP32S2 |
240MHz |
4MB |
320KB |
|
External |
ESP32S2 |
240MHz |
4MB |
320KB |
Fred
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32 |
240MHz |
4MB |
320KB |
Freenove
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
On-board |
ESP32S3 |
240MHz |
8MB |
320KB |
|
External |
ESP32 |
240MHz |
4MB |
320KB |
HONEYLemon
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32 |
240MHz |
4MB |
320KB |
Hardkernel
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
No |
ESP32 |
240MHz |
16MB |
320KB |
Heltec
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32S3 |
240MHz |
8MB |
320KB |
|
External |
ESP32S3 |
240MHz |
8MB |
320KB |
Heltec Automation
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
No |
ESP32 |
240MHz |
4MB |
320KB |
|
No |
ESP32 |
240MHz |
8MB |
320KB |
|
External |
ESP32 |
240MHz |
4MB |
320KB |
|
External |
ESP32 |
240MHz |
8MB |
320KB |
|
External |
ESP32 |
240MHz |
8MB |
320KB |
|
No |
ESP32 |
240MHz |
4MB |
320KB |
Hornbill
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32 |
240MHz |
4MB |
320KB |
|
External |
ESP32 |
240MHz |
4MB |
320KB |
INEX
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
No |
ESP32 |
240MHz |
4MB |
320KB |
Imbrios
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
No |
ESP32 |
240MHz |
4MB |
320KB |
IntoRobot
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
No |
ESP32 |
240MHz |
4MB |
320KB |
IoTaaP
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32 |
240MHz |
4MB |
320KB |
KITS
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
No |
ESP32 |
240MHz |
4MB |
320KB |
Kinetic Dynamics
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32S3 |
240MHz |
4MB |
320KB |
LOGISENSES
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32 |
240MHz |
4MB |
320KB |
Labplus
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
No |
ESP32 |
240MHz |
4MB |
320KB |
LilyGo
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
No |
ESP32 |
240MHz |
4MB |
320KB |
|
External |
ESP32S3 |
240MHz |
16MB |
320KB |
|
External |
ESP32S3 |
240MHz |
4MB |
320KB |
Lion:Bit
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32 |
240MHz |
4MB |
320KB |
|
On-board |
ESP32S3 |
240MHz |
4MB |
320KB |
M5Stack
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32S3 |
240MHz |
8MB |
320KB |
|
No |
ESP32 |
240MHz |
4MB |
320KB |
|
No |
ESP32 |
240MHz |
16MB |
520KB |
|
No |
ESP32 |
240MHz |
16MB |
4.31MB |
|
External |
ESP32S3 |
240MHz |
16MB |
320KB |
|
No |
ESP32 |
240MHz |
16MB |
4.31MB |
|
No |
ESP32 |
240MHz |
16MB |
520KB |
|
External |
ESP32S3 |
240MHz |
8MB |
320KB |
|
No |
ESP32 |
240MHz |
16MB |
4.31MB |
|
No |
ESP32 |
240MHz |
4MB |
320KB |
|
No |
ESP32 |
240MHz |
4MB |
320KB |
|
No |
ESP32 |
240MHz |
4MB |
320KB |
|
No |
ESP32 |
240MHz |
4MB |
320KB |
|
No |
ESP32 |
240MHz |
4MB |
320KB |
MECT SRL
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32S3 |
240MHz |
4MB |
320KB |
|
External |
ESP32S3 |
240MHz |
4MB |
320KB |
MGBOT
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
No |
ESP32 |
240MHz |
4MB |
320KB |
|
No |
ESP32 |
240MHz |
4MB |
320KB |
MH-ET Live
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32 |
240MHz |
4MB |
320KB |
|
External |
ESP32 |
240MHz |
4MB |
320KB |
Magicblocks.io
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
No |
ESP32 |
240MHz |
4MB |
320KB |
MakerAsia
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32 |
240MHz |
4MB |
320KB |
|
No |
ESP32 |
240MHz |
4MB |
320KB |
Microduino
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
No |
ESP32 |
240MHz |
4MB |
320KB |
MotorGo
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32S3 |
240MHz |
4MB |
320KB |
Munich Labs
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32S3 |
240MHz |
8MB |
320KB |
NodeMCU
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32 |
240MHz |
4MB |
320KB |
Noduino
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
No |
ESP32 |
240MHz |
16MB |
320KB |
OLIMEX
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32 |
240MHz |
4MB |
320KB |
|
External |
ESP32 |
240MHz |
4MB |
320KB |
|
External |
ESP32 |
240MHz |
4MB |
320KB |
|
No |
ESP32 |
240MHz |
4MB |
320KB |
|
No |
ESP32 |
240MHz |
4MB |
320KB |
|
No |
ESP32 |
240MHz |
4MB |
320KB |
OROCA
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
No |
ESP32 |
240MHz |
4MB |
320KB |
Onehorse
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
No |
ESP32 |
240MHz |
4MB |
320KB |
PowerFeather
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32S3 |
240MHz |
8MB |
320KB |
ProtoCentral
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
No |
ESP32 |
240MHz |
4MB |
320KB |
Pycom Ltd.
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
No |
ESP32 |
240MHz |
4MB |
320KB |
|
External |
ESP32 |
240MHz |
4MB |
320KB |
|
External |
ESP32 |
240MHz |
4MB |
1.25MB |
|
External |
ESP32 |
240MHz |
4MB |
1.25MB |
Qmobot LLP
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
No |
ESP32 |
240MHz |
4MB |
320KB |
RYMCU
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32C3 |
160MHz |
4MB |
320KB |
|
External |
ESP32 |
240MHz |
4MB |
320KB |
|
On-board |
ESP32S3 |
240MHz |
8MB |
320KB |
RoboHeart
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
No |
ESP32 |
240MHz |
4MB |
320KB |
RoboticsBrno
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32 |
240MHz |
4MB |
320KB |
S.ODI
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
No |
ESP32 |
240MHz |
4MB |
320KB |
SG-O
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32 |
240MHz |
4MB |
320KB |
SQFMI
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32 |
240MHz |
4MB |
320KB |
Seeed Studio
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32S3 |
240MHz |
4MB |
320KB |
|
External |
ESP32C3 |
160MHz |
4MB |
320KB |
|
External |
ESP32S3 |
240MHz |
8MB |
320KB |
Silicognition
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32 |
240MHz |
4MB |
320KB |
Smart Bee
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32S3 |
240MHz |
8MB |
320KB |
|
External |
ESP32S2 |
240MHz |
4MB |
320KB |
|
External |
ESP32C3 |
160MHz |
4MB |
320KB |
|
External |
ESP32S3 |
240MHz |
8MB |
320KB |
|
External |
ESP32S3 |
240MHz |
8MB |
320KB |
SparkFun
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32 |
240MHz |
4MB |
320KB |
|
No |
ESP32 |
240MHz |
4MB |
320KB |
|
External |
ESP32 |
240MHz |
16MB |
320KB |
|
External |
ESP32S2 |
240MHz |
4MB |
320KB |
|
External |
ESP32 |
240MHz |
4MB |
320KB |
SparkFun Electronics
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32 |
240MHz |
4MB |
320KB |
|
External |
ESP32 |
240MHz |
16MB |
320KB |
T3 Foundation
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32 |
240MHz |
4MB |
320KB |
|
External |
ESP32 |
240MHz |
4MB |
320KB |
|
External |
ESP32S3 |
240MHz |
4MB |
320KB |
|
External |
ESP32C3 |
160MHz |
4MB |
320KB |
|
External |
ESP32S2 |
240MHz |
4MB |
320KB |
|
External |
ESP32S2 |
240MHz |
4MB |
320KB |
TAMC
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32 |
240MHz |
8MB |
320KB |
|
External |
ESP32S3 |
240MHz |
8MB |
320KB |
TTGO
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32 |
240MHz |
4MB |
320KB |
|
External |
ESP32 |
240MHz |
4MB |
320KB |
|
External |
ESP32 |
240MHz |
4MB |
320KB |
|
External |
ESP32 |
240MHz |
4MB |
1.25MB |
|
External |
ESP32C3 |
160MHz |
4MB |
320KB |
|
No |
ESP32 |
240MHz |
16MB |
320KB |
|
External |
ESP32 |
240MHz |
4MB |
320KB |
|
No |
ESP32 |
240MHz |
4MB |
1.25MB |
|
External |
ESP32 |
240MHz |
4MB |
1.25MB |
ThaiEasyElec
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32 |
240MHz |
4MB |
320KB |
Trueverit
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32 |
240MHz |
4MB |
320KB |
|
External |
ESP32 |
240MHz |
4MB |
320KB |
|
External |
ESP32 |
240MHz |
4MB |
320KB |
Turta
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
No |
ESP32 |
240MHz |
4MB |
320KB |
Unexpected Maker
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32S2 |
240MHz |
16MB |
320KB |
|
External |
ESP32S2 |
240MHz |
4MB |
320KB |
|
External |
ESP32S3 |
240MHz |
16MB |
320KB |
|
External |
ESP32S3 |
240MHz |
8MB |
320KB |
|
External |
ESP32S3 |
240MHz |
16MB |
320KB |
|
External |
ESP32S2 |
240MHz |
4MB |
320KB |
|
No |
ESP32 |
240MHz |
4MB |
320KB |
|
External |
ESP32S2 |
240MHz |
4MB |
320KB |
|
External |
ESP32S3 |
240MHz |
8MB |
320KB |
University of Sheffield
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32 |
240MHz |
4MB |
320KB |
|
External |
ESP32S3 |
240MHz |
7.94MB |
2.31MB |
|
External |
ESP32S3 |
240MHz |
7.94MB |
8.31MB |
Unknown
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32 |
240MHz |
4MB |
320KB |
Valetron Systems
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32C3 |
160MHz |
4MB |
320KB |
|
External |
ESP32C3 |
160MHz |
4MB |
320KB |
VintLabs
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32 |
240MHz |
4MB |
320KB |
WEMOS
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32 |
240MHz |
4MB |
320KB |
|
External |
ESP32 |
240MHz |
4MB |
320KB |
|
External |
ESP32C3 |
160MHz |
4MB |
320KB |
|
External |
ESP32 |
240MHz |
4MB |
320KB |
|
External |
ESP32 |
240MHz |
4MB |
320KB |
|
External |
ESP32S2 |
240MHz |
4MB |
320KB |
|
External |
ESP32S2 |
240MHz |
4MB |
320KB |
|
External |
ESP32S3 |
240MHz |
16MB |
320KB |
|
External |
ESP32S3 |
240MHz |
4MB |
320KB |
|
External |
ESP32S3 |
240MHz |
16MB |
320KB |
|
External |
ESP32 |
240MHz |
4MB |
320KB |
|
External |
ESP32 |
240MHz |
4MB |
320KB |
|
External |
ESP32 |
240MHz |
4MB |
320KB |
WeAct Studio
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32C3 |
160MHz |
384KB |
400KB |
Widora
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
No |
ESP32 |
240MHz |
16MB |
320KB |
Wireless-Tag
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32 |
240MHz |
4MB |
320KB |
XinaBox
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32 |
240MHz |
4MB |
320KB |
YeaCreate
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
No |
ESP32 |
240MHz |
16MB |
320KB |
microS2
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32S2 |
240MHz |
16MB |
320KB |
oddWires
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32 |
240MHz |
4MB |
320KB |
|
External |
ESP32 |
240MHz |
4MB |
320KB |
senseBox
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32S2 |
240MHz |
4MB |
320KB |
u-blox
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
No |
ESP32 |
240MHz |
2MB |
320KB |
uPesy
Name |
Debug |
MCU |
Frequency |
Flash |
RAM |
---|---|---|---|---|---|
External |
ESP32 |
240MHz |
4MB |
320KB |
|
External |
ESP32 |
240MHz |
4MB |
320KB |